This post will show how to insert Super Column to Cassandra by using Thrift API.
At first, we create a Keyspace named "Sample" and Column Family named "SCTest" via Cassandra-cli.
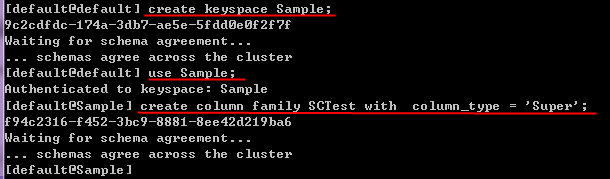
As default , column type was "Standard" , so we need to assign column type to "Super".
then , we using Thrift to insert Super Column to DB , here is the sample code. Following code almost same as Thrift Examples, I only change some place to insert super column.
In the example , I add one column (Key:datetime) with 2 super column , both super column contain different columns.
After insert data success , we can use "List" command to show data in the column family.
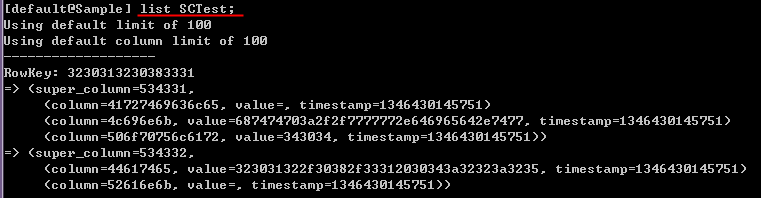
You can see the record added , but it's unreadable . That because Cassandra column default was save as "Byte" , so after convert , the value that we insert was hard to read by human.

We can use "assume" command to make the value readable , after that , you can see the data that we insert was correct , congratulations !!
At first, we create a Keyspace named "Sample" and Column Family named "SCTest" via Cassandra-cli.
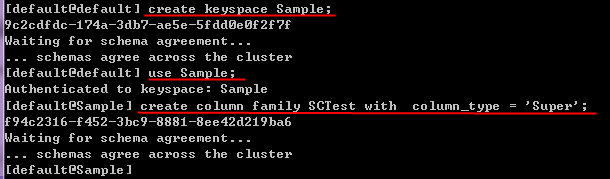
As default , column type was "Standard" , so we need to assign column type to "Super".
then , we using Thrift to insert Super Column to DB , here is the sample code. Following code almost same as Thrift Examples, I only change some place to insert super column.
using System; using System.Collections.Generic; using System.Text; using Apache.Cassandra; using Thrift.Protocol; using Thrift.Transport; namespace Cassandra_SuperColumn { class Program { static void Main() { InsertSuperColumn(); Console.ReadLine(); } protected static void InsertSuperColumn() { //connect to localost cassandra service TTransport frameTransport = new TFramedTransport(new TSocket("localhost", 9160)); TProtocol frameProtocol = new TBinaryProtocol(frameTransport); var client = new Cassandra.Client(frameProtocol, frameProtocol); frameTransport.Open(); Console.WriteLine("Connected to Cassandra"); //set keyspace client.set_keyspace("Sample"); long timeStamp = Convert.ToInt64(DateTime.UtcNow.AddHours(8).Subtract(new DateTime(1970, 1, 1)).TotalMilliseconds); /*SuperColumn group 1*/ //add cols var columns1 = new List<Column> { new Column{Name = Encoding.UTF8.GetBytes("Popular"),Value = Encoding.UTF8.GetBytes("404"),Timestamp = timeStamp}, new Column{Name = Encoding.UTF8.GetBytes("Link"),Value = Encoding.UTF8.GetBytes("http://www.died.tw"),Timestamp = timeStamp}, new Column{Name = Encoding.UTF8.GetBytes("Article"),Value = Encoding.UTF8.GetBytes(""),Timestamp = timeStamp} }; //set super column var superColumn1 = new SuperColumn { Name = Encoding.UTF8.GetBytes("SC1"), Columns = columns1 }; //put into mutation var mu1 = new Mutation { Column_or_supercolumn = new ColumnOrSuperColumn { Super_column = superColumn1 } }; /*SuperColumn group 2*/ var columns2 = new List<Column> { new Column{Name = Encoding.UTF8.GetBytes("Rank"),Value = Encoding.UTF8.GetBytes(""),Timestamp = timeStamp}, new Column{Name = Encoding.UTF8.GetBytes("Date"),Value = Encoding.UTF8.GetBytes(string.Format("{0:yyyy/MM/dd hh:mm:ss}",DateTime.Now)),Timestamp = timeStamp} }; var superColumn2 = new SuperColumn { Name = Encoding.UTF8.GetBytes("SC2"), Columns = columns2 }; var mu2 = new Mutation { Column_or_supercolumn = new ColumnOrSuperColumn { Super_column = superColumn2 } }; //put all mutation to list var lm = new List<Mutation> {mu1,mu2}; //set column family var dictMutation = new Dictionary<string, List<Mutation>> { { "SCTest", lm } }; //set key and input value var insertDataMap = new Dictionary<byte[], Dictionary<string, List<Mutation>>> { { Encoding.UTF8.GetBytes(string.Format("{0:yyyyMMdd}", DateTime.Now)), //key dictMutation //value } }; //batch insert Console.WriteLine("Inserting data"); client.batch_mutate(insertDataMap, ConsistencyLevel.ALL); //close frameTransport.Flush(); frameTransport.Close(); Console.WriteLine("Connect closed"); } } }
In the example , I add one column (Key:datetime) with 2 super column , both super column contain different columns.
After insert data success , we can use "List" command to show data in the column family.
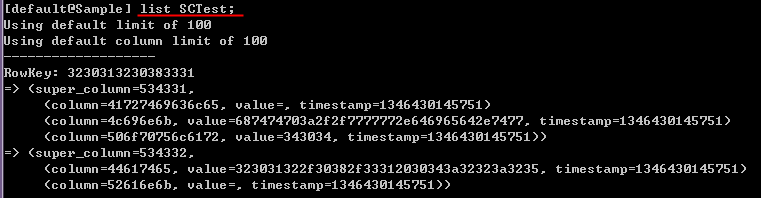
You can see the record added , but it's unreadable . That because Cassandra column default was save as "Byte" , so after convert , the value that we insert was hard to read by human.

We can use "assume" command to make the value readable , after that , you can see the data that we insert was correct , congratulations !!
No comments:
Post a Comment